What are some advanced OOPS interview questions designed for experienced developers and there answer
- Divyansh WsCube
- Nov 4, 2023
- 7 min read
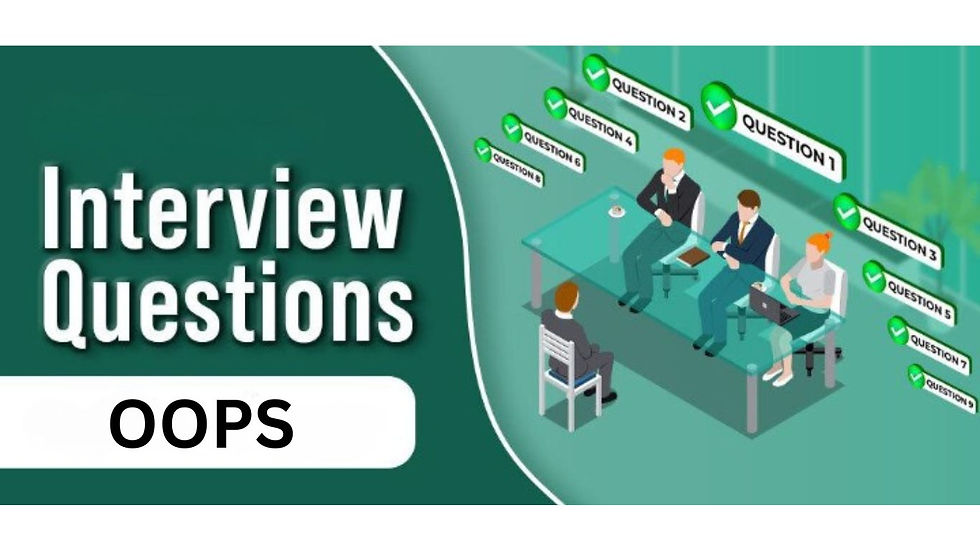
Introduction
Object-Oriented Programming (OOPS) is a fundamental paradigm in software development, and it forms the backbone of modern software design. While basic OOPS principles are well-known, experienced developers may face more advanced OOPS interview questions and answers. These questions delve deeper into design patterns, architectural concepts, and intricate programming scenarios. In this blog, we will explore some advanced OOPS interview questions, along with detailed answers to help experienced developers prepare for challenging interviews.
Question 1: What is the Singleton Pattern, and why is it used in OOPS?
Answer: The Singleton Pattern is a design pattern that restricts the instantiation of a class to a single instance. It is used in OOPS to ensure that a class has only one instance and provides a global point of access to that instance. This pattern is beneficial when exactly one object is needed to coordinate actions across the system. It's commonly used for logging, driver objects, caching, thread pools, database connections, and more.
Question 2: Explain the Factory Method Pattern and its real-world use cases.
Answer: The Factory Method Pattern is a creational design pattern that defines an interface for creating objects but lets subclasses alter the type of objects that will be created. It's used when a class cannot anticipate the type of objects it needs to create. Real-world use cases include document creation (e.g., Word and PDF), GUI frameworks, and framework extensibility.
Question 3: What is the Observer Pattern, and how does it facilitate communication between objects?
Answer: The Observer Pattern is a behavioral design pattern that defines a one-to-many dependency between objects. When the state of one object changes, all its dependents (observers) are notified and updated automatically. It facilitates communication between objects in a loosely coupled manner. For instance, it's used in implementing event handling systems, GUI frameworks, and distributed systems where multiple components need to react to state changes.
Question 4: Describe the Decorator Pattern and its role in enhancing object functionality.
Answer: The Decorator Pattern is a structural design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. It's used to extend object functionality in a flexible way. For example, in GUI frameworks, decorators can add features like borders or scrollbars to a base component.
Question 5: What is Dependency Injection, and how does it improve code maintainability?
Answer: Dependency Injection is a software design pattern that helps implement the Inversion of Control (IoC) principle. It involves supplying the dependencies of an object from the outside, rather than creating them within the object. This improves code maintainability by decoupling components and making them easier to test and change. Dependency Injection frameworks like Spring in Java exemplify this pattern.
Question 6: Explain the SOLID principles in OOPS and provide examples for each.
Answer: The SOLID principles are a set of five principles for software design:
Single Responsibility Principle (SRP): A class should have only one reason to change. For example, a class that handles both logging and data processing violates SRP.
Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification. For example, using interfaces and abstract classes to add new functionality without modifying existing code.
Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types. For example, a derived class should be able to replace its base class without affecting program correctness.
Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they don't use. For example, breaking down large interfaces into smaller, focused ones.
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules, but both should depend on abstractions. For example, using interfaces to decouple high-level and low-level modules.
Question 7: What are design patterns and their role in software development?
Answer: Design patterns are proven solutions to recurring design problems in software development. They provide templates for structuring code to solve common issues efficiently. Their role includes improving code maintainability, extensibility, and reducing development time. Some famous design patterns include Singleton, Factory Method, Observer, and Decorator, which were discussed earlier.
Question 8: What is the role of the Service Locator Pattern, and when should it be used?
Answer: The Service Locator Pattern is a design pattern used to encapsulate the processes involved in obtaining a service with a strong reference to a central registry. It should be used in scenarios where objects require access to services with a minimum of fuss. For example, it's commonly used in enterprise applications where multiple services need to be located and accessed.
Question 9: What is Aspect-Oriented Programming (AOP), and how does it complement OOPS?
Answer: Aspect-Oriented Programming is a paradigm that complements OOPS by separating concerns that cross-cut an application, such as logging, security, and transactions, from the core business logic. AOP allows for the modularization of cross-cutting concerns, making code cleaner and more maintainable. Frameworks like Spring AOP in Java implement AOP principles.
Question 10: Explain the concept of a "Virtual Proxy" in the Proxy Pattern and provide a real-world example.
Answer: In the Proxy Pattern, a "Virtual Proxy" is a placeholder for an expensive-to-create object. It allows you to create the object only when it's required. For example, in a multimedia application, a virtual proxy can represent a high-resolution image that is loaded on-demand when the user zooms in, saving memory and enhancing performance.
Also Read: What are the toughest HTML interview questions you've ever encountered, and how did you answer them?
Question 11: Discuss the role of the Command Pattern in decoupling sender and receiver in software design.
Answer: The Command Pattern is a behavioral design pattern that decouples the sender and receiver of a request. It does this by encapsulating the request as an object, thereby allowing you to parameterize clients with queues, requests, and operations. This decoupling is beneficial in scenarios where requests need to be queued, stored, and executed at different times.
Question 12: What is the role of the Memento Pattern in capturing an object's internal state?
Answer: The Memento Pattern is a behavioral design pattern that allows the externalization of an object's internal state. It provides the ability to capture an object's state, save it, and restore it later. This pattern is particularly useful in scenarios like undo/redo functionality, where you need to save and restore the previous state of an object.
Question 13: Explain the Adapter Pattern and provide an example of its usage in real-world scenarios.
Answer: The Adapter Pattern is a structural design pattern that allows the interface of an existing class to be used as another interface. It's used when you want to make existing classes work with others without modifying their source code. A common real-world example is using adapters to connect various electronic devices to power outlets with different plug types.
Question 14: What is the Flyweight Pattern, and how does it save memory in software design?
Answer: The Flyweight Pattern is a structural design pattern that minimizes memory usage or computational expenses by sharing as much as possible with similar objects. It's particularly useful in scenarios where a large number of similar objects need to be created. For instance, in a text editor, the Flyweight Pattern can be used to share font and formatting information among characters in the document.
Question 15: Describe the Bridge Pattern and how it separates an object's abstraction from its implementation.
Answer: The Bridge Pattern is a structural design pattern that separates the abstraction from its implementation so that both can vary independently. It's used when you want to avoid a permanent binding between an abstraction and its implementation, allowing them to change without affecting each other. An example is a remote control where the Bridge Pattern separates the device's abstract controls from its concrete implementation.
Question 16: How does the Strategy Pattern facilitate dynamic algorithm selection and switching?
Answer: The Strategy Pattern is a behavioral design pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows clients to choose an algorithm from a family of algorithms dynamically. In scenarios where an application requires dynamic algorithm selection and switching, the Strategy Pattern is a useful solution.
Question 17: Explain the Composite Pattern and its use in creating complex structures.
Answer: The Composite Pattern is a structural design pattern that lets you compose objects into a tree structure to represent part-whole hierarchies. It's used when clients need to treat individual objects and compositions of objects uniformly. For example, in a graphics application, both shapes (individual objects) and compound shapes (compositions) can be manipulated uniformly using the Composite Pattern.
Question 18: How does the Chain of Responsibility Pattern facilitate the passing of requests among handlers?
Answer: The Chain of Responsibility Pattern is a behavioral design pattern that passes a request along a chain of handlers. Each handler decides either to process the request or pass it to the next handler in the chain. It's useful in scenarios where there are multiple handlers for a request, and the specific handler is determined at runtime. For example, in a logging system, different loggers can be part of a chain to handle log entries based on their severity.
Question 19: Describe the Proxy Pattern and its different types (e.g., Virtual Proxy, Remote Proxy).
Answer: The Proxy Pattern is a structural design pattern that provides a surrogate or placeholder for another object to control access to it. Types of proxies include:
Virtual Proxy: A placeholder for an expensive-to-create object.
Remote Proxy: Represents an object in a different address space, such as in remote method invocation scenarios.
Cache Proxy: Manages the caching of a resource to improve performance.
Smart Proxy: Adds functionality like reference counting and lazy initialization.
Question 20: How does the Mediator Pattern centralize complex communications and interactions between objects?
Answer: The Mediator Pattern is a behavioral design pattern that defines an object that centralizes communication between other objects. It promotes loose coupling by ensuring that objects do not need to be aware of each other's existence. In a chat application, for instance, a mediator can manage messages between users, ensuring that each user doesn't need to know the specifics of other users' implementations.
Conclusion
In the world of software development, advanced OOPS concepts and design patterns play a pivotal role in solving complex problems, enhancing code quality, and creating maintainable and extensible software systems. Experienced developers can greatly benefit from understanding these advanced OOPS principles and patterns, as they provide solutions to intricate design challenges. Preparing for these advanced OOPS interview questions and mastering their application can open doors to exciting and rewarding career opportunities in software development.
Comments